Optimizing Database Operations in Django
- General
- Performance and Security
- Quality Engineering
- User Experience & Design
Optimizing Database Operations in Django
Why do you need to know this?
Optimizing database operations is crucial for improved application performance, scalability, cost efficiency, and enhanced user experience. It reduces query execution time, minimizes resource utilization and ensures the database can handle increasing data volume and user traffic. Optimization leads to faster load times, smoother interactions, and lower infrastructure costs. By optimizing the database, developers can create applications that deliver optimal performance, scale effectively, and provide a seamless user experience.
In this blog, we will explore some essential tips and practices that can help us to optimizing database operations
And Best practices
1. Use Selective Field Retrieval
When fetching data from the database, retrieve only the necessary fields using the values() or values_list() methods. This reduces the amount of data transferred between the database and Django, resulting in improved performance.
2. Limit Query Results:
Retrieve only the required number of records instead of retrieving the entire dataset. This approach minimizes the impact on performance. Use methods such as limit() or slicing ([:n]).
3. Utilize select_related() and prefetch_related()
These methods optimize query performance by reducing the number of database queries. Use select_related() to fetch related objects in a single query and prefetch_related() to fetch related objects efficiently, particularly when dealing with large datasets.
For further details, kindly refer to Prefetch_related and select_related functions in django
4. Database Indexing
Identify frequently used fields in your queries and apply appropriate database indexes. Indexing improves query performance by reducing the time taken to find matching records. Django provides support for defining indexes on model fields, leveraging the power of database indexing.
5. Mitigate the N+1 Query Problem
Be mindful of the N+1 query problem, which occurs when a query is executed for each related object individually. Address this issue by utilizing select_related() or prefetch_related() to fetch related objects in an optimized manner, reducing the number of database queries.
6. Leverage Database-specific Optimization Techniques
Different databases offer unique optimization techniques. Understand the database engine used by our Django project (e.g., PostgreSQL, MySQL, SQLite) and explore database-specific optimizations such as database-specific indexes or query hints. Utilizing these techniques can significantly enhance performance. These optimizations take advantage of the unique capabilities and functionalities provided by the DBMS.
Here are some examples of database-specific optimizations:
- Query Optimization: DBMSs employ various query optimization techniques to improve the execution of database queries. These techniques include query rewriting, query plan optimization, cost-based optimization, and index selection. The specific algorithms and optimization strategies used may vary across different DBMSs.
- Indexing: Different DBMSs offer various types of indexes, such as B-tree, hash, or bitmap indexes. Choosing the appropriate index type based on the query patterns and data characteristics can significantly improve query performance.
- Partitioning: Partitioning involves dividing a large table into smaller, more manageable partitions based on specific criteria such as range, list, or hash partitioning. Partitioning can enhance query performance by allowing the DBMS to perform operations on a smaller subset of data.
- Materialized Views: Materialized views are precomputed views stored as physical tables. They are updated periodically or incrementally, resulting in faster query performance by avoiding complex computations. DBMSs may provide features for creating and maintaining materialized views efficiently.
- Database Caching: Many DBMSs include caching mechanisms that allow frequently accessed data to be stored in memory. Caching can reduce disk I/O and improve response times for read-intensive workloads.
- Stored Procedures and Functions: DBMSs often provide the ability to define and execute stored procedures and functions. These database-specific program units can improve performance by executing complex operations on the server side, reducing network overhead.
- Database Configuration and Tuning: Each DBMS has its own set of configuration parameters and settings that can be adjusted to optimize performance. Tuning database configuration parameters, such as buffer sizes, connection pool sizes, and concurrency settings, can have a significant impact on overall performance.
7. Consider Denormalization
Denormalization is technique where redundant or duplicate data is intentionally introduced into the database schema. This deviation from normalization principles is done to optimize query performance and improve overall system performance. The goal is to improve query performance by reducing the number of joins and simplifying complex queries. Denormalization is typically employed in read-heavy systems, where the need for faster query execution outweighs the need for strict data consistency and update efficiency.
There are several denormalization techniques:
- Flattening: Combining multiple related tables into a single table by including all relevant columns in one denormalized table. This reduces the need for joins and improves query performance.
- Duplication: Duplicating data from one table into another table to eliminate the need for joining tables. This redundancy allows for faster retrieval at the expense of increased storage space.
- Pre-joining: Storing pre-joined data in a denormalized table to avoid the need for joining multiple tables during query execution. This technique can speed up complex queries with frequent joins.
Denormalization should be used judiciously and carefully. It is generally applied in situations where read performance is critical, and careful consideration is given to handle data updates to maintain consistency across denormalized tables. It’s worth noting that denormalization is not a one-size-fits-all solution. Each application has its own unique requirements and performance characteristics, so it’s essential to analyze the specific use cases, query patterns, and system performance requirements before deciding to denormalize the database schema.
8. Monitor and Analyze Database Performance
Regularly monitor the performance of your database using tools like Django Debug Toolbar or database-specific monitoring tools. Identify slow queries, analyze their execution plans, and optimize them accordingly. Monitoring performance helps identify bottlenecks and ensures continuous improvement.
9. Caching
Leverage caching techniques to store frequently accessed data in memory. Django provides various caching backends such as Memcached or Redis. Cache data that doesn’t change frequently to reduce the number of database hits, resulting in improved response times.
Caching can be beneficial in Django applications in various scenarios. Here are some common situations where you might consider using caching:
- Frequently Accessed Data: If our application accesses data from a database or performs expensive calculations that are requested frequently, caching can help improve performance.
- Expensive Database Queries: If you have complex database queries that require joining multiple tables, aggregations, or involve heavy computations, caching the query results can significantly reduce the load on your database. Instead of executing the query each time, you can cache the results and serve them from the cache for subsequent requests.
- Static Content: If your application serves static content like “Terms and Conditions” data or logo image names etc, caching them can enhance performance.
- Dynamic Content with Low Update Frequency: If you have dynamic content that doesn’t change frequently, caching can be beneficial. For example, if you have sections of your website that display data such as “Popular Posts” or “Trending Products,” you can cache the results for a certain duration and refresh the cache periodically. This approach reduces the load on your back-end and improves response times for users.
- Heavy Computation or Rendering: If your application performs computationally intensive operations or generates complex views or reports, caching the results can save processing time.
10. Load Testing and Profiling
Perform load testing on your application to identify performance bottlenecks is also help us in optimizing database operations. Utilize profiling tools like Django Silk or Django DevServer to identify areas of code that require optimization. Profiling helps pinpoint specific functions or queries that may be causing performance issues. Here is a list of popular tools for load testing and profiling Django applications:
- Apache JMeter
- Locust
- Siege
- Django Debug Toolbar
- Django Silk
- New Relic
- Py-Spy
These tools can assist us in load testing our Django application to determine how it performs under different levels of traffic and also help us identify performance issues and bottlenecks during the profiling process. Remember to carefully analyze the results and optimize our code and database queries based on the findings to improve the overall performance of our Django application.
Concluding
Optimizing database operations is crucial for the performance and scalability of Django applications. By implementing these best practices and tips, we can significantly enhance the efficiency of their database operations. From selective field retrieval to utilizing caching and employing database-specific optimizations, each technique plays a vital role in achieving optimal performance. Regular monitoring, profiling, and load testing ensure that the application continues to deliver the best performance possible. By following these guidelines, Django developers can create high-performing applications that scale effectively.
Remember, optimizing database operations is an ongoing process. As your application evolves, review and analyze your queries regularly, and adapt your optimization strategies accordingly.
Recommended Link: https://docs.djangoproject.com/en/4.2/topics/db/optimization/
If you enjoyed this article, share it with your friends and colleagues!
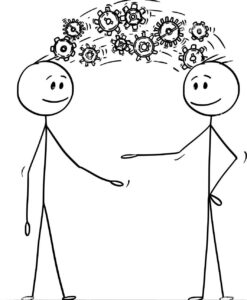
Optimize Database Operations in Django